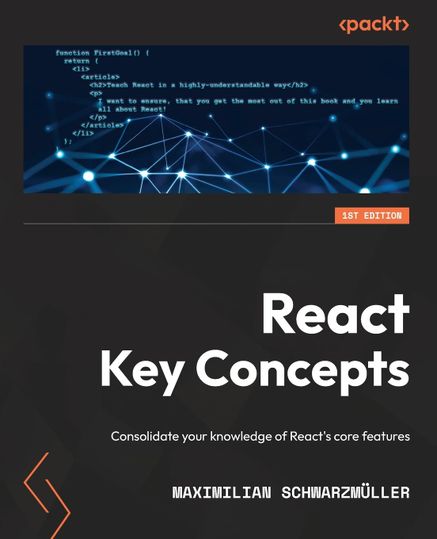
React
React Key Concepts: Consolidate your knowledge of React's core features
Maximilian Schwarzmüller (, 2022
Inhaltsverzeichnis des Buches
- React Key Concepts
- Preface
- About the Book
- About the Author
- Audience
- Prospective Table of Contents
- Conventions
- Setting Up Your Environment
- Installing React.js
- Downloading the Code Bundle
- Get in Touch
- Please Leave a Review
- Download A Free PDF Copy Of This Book
- 1. React – What and Why
- Introduction
- What Is React?
- The Problem with "Vanilla JavaScript"
- React and Declarative Code
- How React Manipulates the DOM
- Introducing Single Page Applications
- Creating a React Project
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- 2. Understanding React Components and JSX
- Introduction
- What Are Components?
- Why Components?
- The Anatomy of a Component
- What Exactly Are Component Functions?
- What Does React Do with All These Components?
- Built-in Components
- Naming Conventions
- JSX vs HTML vs Vanilla JavaScript
- Using React without JSX
- JSX Elements Are Treated like Regular JavaScript Values
- JSX Elements Must Be Self-Closing
- Outputting Dynamic Content
- When Should You Split Components?
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 2.1: Creating a React App to Present Yourself
- Activity 2.2: Creating a React App to Log Your Goals for This Book
- 3. Components and Props
- Introduction
- Not There Yet
- Using Props in Components
- Passing Props to Components
- Consuming Props in a Component
- Components, Props, and Reusability
- The Special "children" Prop
- Which Components Need Props?
- How to Deal with Multiple Props
- Spreading Props
- Prop Chains/Prop Drilling
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 3.1: Creating an App to Output Your Goals for This Book
- 4. Working with Events and State
- Introduction
- What's the Problem?
- How Not to Solve the Problem
- A Better Incorrect Solution
- Properly Reacting to Events
- Updating State Correctly
- A Closer Look at useState()
- A Look under the Hood of React
- Naming Conventions
- Allowed State Value Types
- Working with Multiple State Values
- Using Multiple State Slices
- Managing Combined State Objects
- Updating State Based on Previous State Correctly
- Two-Way Binding
- Deriving Values from State
- Working with Forms and Form Submission
- Lifting State Up
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 4.1: Building a Simple Calculator
- Activity 4.2: Enhancing the Calculator
- 5. Rendering Lists and Conditional Content
- Introduction
- What Are Conditional Content and List Data?
- Rendering Content Conditionally
- Different Ways of Rendering Content Conditionally
- Utilizing Ternary Expressions
- Abusing JavaScript Logical Operators
- Get Creative!
- Which Approach Is Best?
- Setting Element Tags Conditionally
- Outputting List Data
- Mapping List Data
- Updating Lists
- A Problem with List Items
- Keys to the Rescue!
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 5.1: Showing a Conditional Error Message
- Activity 5.2: Outputting a List of Products
- 6. Styling React Apps
- Introduction
- How Does Styling Work in React Apps?
- Using Inline Styles
- Setting Styles via CSS Classes
- Setting Styles Dynamically
- Conditional Styles
- Combining Multiple Dynamic CSS Classes
- Merging Multiple Inline Style Objects
- Building Components with Customizable Styles
- Customization with Fixed Configuration Options
- The Problem with Unscoped Styles
- Scoped Styles with CSS Modules
- The styled-components Library
- Using Other CSS or JavaScript Styling Libraries and Frameworks
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 6.1: Providing Input Validity Feedback upon Form Submission
- Activity 6.2: Using CSS Modules for Style Scoping
- 7. Portals and Refs
- Introduction
- A World without Refs
- Refs versus State
- Using Refs for More than DOM Access
- Forwarding Refs
- Controlled versus Uncontrolled Components
- React and Where Things End up in the DOM
- Portals to the Rescue
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Have Learned
- Activity 7.1: Extract User Input Values
- Activity 7.2: Add a Side-Drawer
- 8. Handling Side Effects
- Introduction
- What's the Problem?
- Understanding Side Effects
- Side Effects Are Not Just about HTTP Requests
- Dealing with Side Effects with the useEffect() Hook
- How to Use useEffect()
- Effects and Their Dependencies
- Unnecessary Dependencies
- Cleaning Up after Effects
- Dealing with Multiple Effects
- Functions as Dependencies
- Avoiding Unnecessary Effect Executions
- Effects and Asynchronous Code
- Rules of Hooks
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 8.1: Building a Basic Blog
- 9. Behind the Scenes of React and Optimization Opportunities
- Introduction
- Revisiting Component Evaluations and Updates
- What Happens When a Component Function Is Called
- The Virtual DOM vs the Real DOM
- State Batching
- Avoiding Unnecessary Child Component Evaluations
- Avoiding Costly Computations
- Utilizing useCallback()
- Avoiding Unnecessary Code Download
- Reducing Bundle Sizes via Code Splitting (Lazy Loading)
- Strict Mode
- Debugging Code and the React Developer Tools
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 9.1: Optimize an Existing App
- 10. Working with Complex State
- Introduction
- A Problem with Cross-Component State
- Using Context to Handle Multi-Component State
- Providing and Managing Context Values
- Using Context in Nested Components
- Changing Context from Nested Components
- Getting Better Code Completion
- Context or "Lifting State Up"?
- Outsourcing Context Logic into Separate Components
- Combining Multiple Contexts
- Limitations of useState( )
- Managing State with useReducer( )
- Understanding Reducer Functions
- Dispatching Actions
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 10.1: Migrating an App to the Context API
- Activity 10.2: Replacing useState() with useReducer()
- 11. Building Custom React Hooks
- Introduction
- Why Would You Build Custom Hooks?
- What Are Custom Hooks?
- A First Custom Hook
- Custom Hooks: A Flexible Feature
- Custom Hooks and Parameters
- Custom Hooks and Return Values
- A More Complex Example
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 11.1: Build a Custom Keyboard Input Hook
- 12. Multipage Apps with React Router
- Introduction
- One Page Is Not Enough
- Getting Started with React Router and Defining Routes
- Adding Page Navigation
- From Link to NavLink
- Route Components versus "Normal" Components
- From Static to Dynamic Routes
- Extracting Route Parameters
- Creating Dynamic Links
- Navigating Programmatically
- Redirecting
- Nested Routes
- Handling Undefined Routes
- Lazy Loading
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 12.1: Creating a Basic Three-Page Website
- Activity 12.2: Enhancing the Basic Website
- 13. Managing Data with React Router
- Introduction
- Data Fetching and Routing Are Tightly Coupled
- Sending HTTP Requests without React Router
- Loading Data with React Router
- Enabling These Extra Router Features
- Loading Data for Dynamic Routes
- Loaders, Requests, and Client-Side Code
- Layouts Revisited
- Reusing Data across Routes
- Handling Errors
- Onward to Data Submission
- Working with action() and Form Data
- Returning Data Instead of Redirecting
- Controlling Which <Form> Triggers Which Action
- Reflecting the Current Navigation Status
- Submitting Forms Programmatically
- Behind-the-Scenes Data Fetching and Submission
- Deferring Data Loading
- Summary and Key Takeaways
- What's Next?
- Test Your Knowledge!
- Apply What You Learned
- Activity 13.1: A To-Dos App
- 14. Next Steps and Further Resources
- Introduction
- How Should You Proceed?
- Interesting Problems to Explore
- Build a Shopping Cart
- Build an Application's Authentication System (User Signup and Login)
- Build an Event Management Website
- Common and Popular React Libraries
- Other Resources
- Beyond React for Web Applications
- Final Words
- Appendix