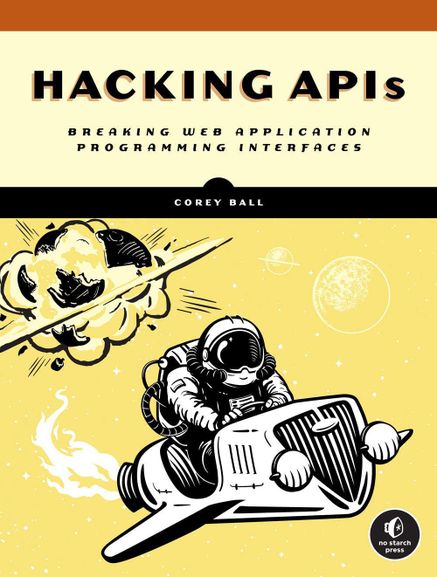
API
Web Security
Hacking APIs: Breaking Web Application Programming Interfaces
Corey Ball, 2022
Inhaltsverzeichnis des Buches
- Praise for Hacking APIs
- Title Page
- Copyright
- Dedication
- About the Author
- Foreword
- Acknowledgments
- Introduction
- The Allure of Hacking Web APIs
- This Book’s Approach
- Hacking the API Restaurant
- Part I: How Web API Security Works
- Chapter 0: Preparing for Your Security Tests
- Receiving Authorization
- Threat Modeling an API Test
- Which API Features You Should Test
- API Authenticated Testing
- Web Application Firewalls
- Mobile Application Testing
- Auditing API Documentation
- Rate Limit Testing
- Restrictions and Exclusions
- Security Testing Cloud APIs
- DoS Testing
- Reporting and Remediation Testing
- A Note on Bug Bounty Scope
- Summary
- Chapter 1: How Web Applications Work
- Web App Basics
- The URL
- HTTP Requests
- HTTP Responses
- HTTP Status Codes
- HTTP Methods
- Stateful and Stateless HTTP
- Web Server Databases
- SQL
- NoSQL
- How APIs Fit into the Picture
- Summary
- Chapter 2: The Anatomy of Web APIs
- How Web APIs Work
- Standard Web API Types
- RESTful APIs
- GraphQL
- REST API Specifications
- API Data Interchange Formats
- JSON
- XML
- YAML
- API Authentication
- Basic Authentication
- API Keys
- JSON Web Tokens
- HMAC
- OAuth 2.0
- No Authentication
- APIs in Action: Exploring Twitter’s API
- Summary
- Chapter 3: Common API Vulnerabilities
- Information Disclosure
- Broken Object Level Authorization
- Broken User Authentication
- Excessive Data Exposure
- Lack of Resources and Rate Limiting
- Broken Function Level Authorization
- Mass Assignment
- Security Misconfigurations
- Injections
- Improper Assets Management
- Business Logic Vulnerabilities
- Summary
- Part II: Building an API Testing Lab
- Chapter 4: Your API Hacking System
- Kali Linux
- Analyzing Web Apps with DevTools
- Capturing and Modifying Requests with Burp Suite
- Setting Up FoxyProxy
- Adding the Burp Suite Certificate
- Navigating Burp Suite
- Intercepting Traffic
- Altering Requests with Intruder
- Crafting API Requests in Postman, an API Browser
- The Request Builder
- Environments
- Collections
- The Collection Runner
- Code Snippets
- The Tests Panel
- Configuring Postman to Work with Burp Suite
- Supplemental Tools
- Performing Reconnaissance with OWASP Amass
- Discovering API Endpoints with Kiterunner
- Scanning for Vulnerabilities with Nikto
- Scanning for Vulnerabilities with OWASP ZAP
- Fuzzing with Wfuzz
- Discovering HTTP Parameters with Arjun
- Summary
- Lab #1: Enumerating the User Accounts in a REST API
- Chapter 5: Setting Up Vulnerable API Targets
- Creating a Linux Host
- Installing Docker and Docker Compose
- Installing Vulnerable Applications
- The completely ridiculous API (crAPI)
- OWASP DevSlop’s Pixi
- OWASP Juice Shop
- Damn Vulnerable GraphQL Application
- Adding Other Vulnerable Apps
- Hacking APIs on TryHackMe and HackTheBox
- Summary
- Lab #2: Finding Your Vulnerable APIs
- Part III: Attacking APIs
- Chapter 6: Discovery
- Passive Recon
- The Passive Recon Process
- Google Hacking
- ProgrammableWeb’s API Search Directory
- Shodan
- OWASP Amass
- Exposed Information on GitHub
- Active Recon
- The Active Recon Process
- Baseline Scanning with Nmap
- Finding Hidden Paths in Robots.txt
- Finding Sensitive Information with Chrome DevTools
- Validating APIs with Burp Suite
- Crawling URIs with OWASP ZAP
- Brute-Forcing URIs with Gobuster
- Discovering API Content with Kiterunner
- Summary
- Lab #3: Performing Active Recon for a Black Box Test
- Chapter 7: Endpoint Analysis
- Finding Request Information
- Finding Information in Documentation
- Importing API Specifications
- Reverse Engineering APIs
- Adding API Authentication Requirements to Postman
- Analyzing Functionality
- Testing Intended Use
- Performing Privileged Actions
- Analyzing API Responses
- Finding Information Disclosures
- Finding Security Misconfigurations
- Verbose Errors
- Poor Transit Encryption
- Problematic Configurations
- Finding Excessive Data Exposures
- Finding Business Logic Flaws
- Summary
- Lab #4: Building a crAPI Collection and Discovering Excessive Data Exposure
- Chapter 8: Attacking Authentication
- Classic Authentication Attacks
- Password Brute-Force Attacks
- Password Reset and Multifactor Authentication Brute-Force Attacks
- Password Spraying
- Including Base64 Authentication in Brute-Force Attacks
- Forging Tokens
- Manual Load Analysis
- Live Token Capture Analysis
- Brute-Forcing Predictable Tokens
- JSON Web Token Abuse
- Recognizing and Analyzing JWTs
- The None Attack
- The Algorithm Switch Attack
- The JWT Crack Attack
- Summary
- Lab #5: Cracking a crAPI JWT Signature
- Chapter 9: Fuzzing
- Effective Fuzzing
- Choosing Fuzzing Payloads
- Detecting Anomalies
- Fuzzing Wide and Deep
- Fuzzing Wide with Postman
- Fuzzing Deep with Burp Suite
- Fuzzing Deep with Wfuzz
- Fuzzing Wide for Improper Assets Management
- Testing Request Methods with Wfuzz
- Fuzzing “Deeper” to Bypass Input Sanitization
- Fuzzing for Directory Traversal
- Summary
- Lab #6: Fuzzing for Improper Assets Management Vulnerabilities
- Chapter 10: Exploiting Authorization
- Finding BOLAs
- Locating Resource IDs
- A-B Testing for BOLA
- Side-Channel BOLA
- Finding BFLAs
- A-B-A Testing for BFLA
- Testing for BFLA in Postman
- Authorization Hacking Tips
- Postman’s Collection Variables
- Burp Suite Match and Replace
- Summary
- Lab #7: Finding Another User’s Vehicle Location
- Chapter 11: Mass Assignment
- Finding Mass Assignment Targets
- Account Registration
- Unauthorized Access to Organizations
- Finding Mass Assignment Variables
- Finding Variables in Documentation
- Fuzzing Unknown Variables
- Blind Mass Assignment Attacks
- Automating Mass Assignment Attacks with Arjun and Burp Suite Intruder
- Combining BFLA and Mass Assignment
- Summary
- Lab #8: Changing the Price of Items in an Online Store
- Chapter 12: Injection
- Discovering Injection Vulnerabilities
- Cross-Site Scripting (XSS)
- Cross-API Scripting (XAS)
- SQL Injection
- Manually Submitting Metacharacters
- SQLmap
- NoSQL Injection
- Operating System Command Injection
- Summary
- Lab #9: Faking Coupons Using NoSQL Injection
- Part IV: Real-World API Hacking
- Chapter 13: Applying Evasive Techniques and Rate Limit Testing
- Evading API Security Controls
- How Security Controls Work
- API Security Control Detection
- Using Burner Accounts
- Evasive Techniques
- Automating Evasion with Burp Suite
- Automating Evasion with Wfuzz
- Testing Rate Limits
- A Note on Lax Rate Limits
- Path Bypass
- Origin Header Spoofing
- Rotating IP Addresses in Burp Suite
- Summary
- Chapter 14: Attacking GraphQL
- GraphQL Requests and IDEs
- Active Reconnaissance
- Scanning
- Viewing DVGA in a Browser
- Using DevTools
- Reverse Engineering the GraphQL API
- Directory Brute-Forcing for the GraphQL Endpoint
- Cookie Tampering to Enable the GraphiQL IDE
- Reverse Engineering the GraphQL Requests
- Reverse Engineering a GraphQL Collection Using Introspection
- GraphQL API Analysis
- Crafting Requests Using the GraphiQL Documentation Explorer
- Using the InQL Burp Extension
- Fuzzing for Command Injection
- Summary
- Chapter 15: Data Breaches and Bug Bounties
- The Breaches
- Peloton
- USPS Informed Visibility API
- T-Mobile API Breach
- The Bounties
- The Price of Good API Keys
- Private API Authorization Issues
- Starbucks: The Breach That Never Was
- An Instagram GraphQL BOLA
- Summary
- Conclusion
- Appendix A: API Hacking Checklist
- Appendix B: Additional Resources
- Index