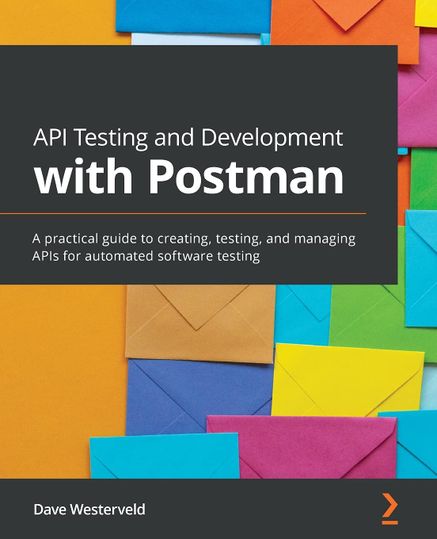
API
Software-Testing
API Testing and Development with Postman: A practical guide to creating, testing, and managing APIs for automated software testing
Dave Westerveld, 2021
Inhaltsverzeichnis des Buches
- Contributors
- About the author
- About the reviewer
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Download the example code files
- Download the color images
- Conventions used
- Get in touch
- Reviews
- Section 1: API Testing Theory and Terminology
- Chapter 1: API Terminology and Types
- What is an API?
- Types of API calls
- Installing Postman
- Starting Postman
- Setting up a request in Postman
- The structure of an API request
- API endpoints
- API actions
- API parameters
- API headers
- API body
- API response
- Learning by doing – making API calls
- Considerations for API testing
- Beginning with exploration
- Looking for business problems
- Trying weird things
- Different types of APIs
- REST APIs
- SOAP APIs
- GraphQL APIs
- GraphQL API example
- Summary
- Chapter 2: Principles of API Design
- Technical requirements
- Start with the purpose
- Figuring out the purpose
- Creating usable APIs
- Usable API structure
- Good error messages
- Document your API
- Documenting with Postman
- Good practices for API documentation
- RESTful API Modeling Language
- Designing an API
- Case study – Designing an e-commerce API
- Modeling an existing API design
- Summary
- Chapter 3: OpenAPI and API Specifications
- Technical requirements
- What are API specifications?
- API specification terminology
- Types of API specifications
- Creating OASes
- Parts of an OAS
- Defining API schema
- Using API specifications in Postman
- Creating mocks from an OAS
- Creating tests from an OAS
- Summary
- Chapter 4: Considerations for Good API Test Automation
- Technical requirements
- Exploring and automating
- Exercise – considerations for good API test automation
- Writing good automation
- Types of API tests
- Organizing and structuring tests
- Creating the test structure
- Organizing the tests
- Creating maintainable tests
- Using logging
- Test reports
- Summary
- Section 2: Using Postman When Working with an Existing API
- Chapter 5: Understanding Authorization Options
- Understanding API security
- Authorization in APIs
- Authentication in APIs
- API security in Postman
- Getting started with authorization in Postman
- Using Basic Auth
- Using Bearer Tokens
- Using API keys
- Using AWS Signature
- Using OAuth
- Using Digest auth and Hawk authentication
- Using NTLM authentication
- Using Akamai EdgeGrid
- Security testing with Postman
- Fuzzing
- Command injection
- Authorization testing
- Integrating with Burp Suite
- Summary
- Chapter 6: Creating Test Validation Scripts
- Technical requirements
- Checking API responses
- Checking the status code in a response
- Checking the body of a response
- Checking headers
- Custom assertion objects in Postman
- Creating your own tests
- Creating folder and collection tests
- Cleaning up after tests
- Setting up pre-request scripts
- Using variables in pre-request scripts
- Passing data between tests
- Building request workflows
- Using environments in Postman
- Managing environment variables
- Editing environment variables
- Summary
- Chapter 7: Data-Driven Testing
- Technical requirements
- Defining data-driven testing
- Setting up data-driven inputs
- Thinking about the outputs for data-driven tests
- Creating a data-driven test in Postman
- Creating the data input
- Adding a test
- Comparing responses to data from a file
- Challenge – data-driven testing with multiple APIs
- Challenge setup
- Challenge hints
- Summary
- Chapter 8: Running API Tests in CI with Newman
- Technical requirements
- Getting Newman set up
- Installing Newman
- Running Newman
- Understanding Newman run options
- Using environments in Newman
- Running data-driven tests in Newman
- Other Newman options
- Reporting on tests in Newman
- Using Newman's built-in reporters
- Using external reporters
- Creating your own reporter
- Integrating Newman into CI builds
- General principles for using Newman in CI builds
- Example of using Travis CI
- Summary
- Chapter 9: Monitoring APIs with Postman
- Technical requirements
- Setting up a monitor in Postman
- Creating a monitor
- Using additional monitor settings
- Adding tests to a monitor
- Viewing monitor results
- Cleaning up the monitors
- Summary
- Chapter 10: Testing an Existing API
- Technical requirements
- Finding bugs in an API
- Setting up a local API for testing
- Testing the API
- Finding bugs in the API
- Resetting the service
- Example bug
- Automating API tests
- Reviewing API automation ideas
- Setting up a collection in Postman
- Creating the tests
- An example of automated API tests
- Setting up a collection in Postman
- Creating the tests
- Sharing your work
- Sharing a collection in Postman
- Summary
- Section 3: Using Postman to Develop an API
- Chapter 11: Designing an API Specification
- Technical requirements
- Creating an API specification
- Starting the file
- Defining the endpoints
- Defining parameters
- Adding additional responses
- Describing request bodies
- Using examples
- Adding the API to Postman
- Contract testing
- Understanding API versioning
- Thinking through when to create a new API version
- Summary
- Chapter 12: Creating and Using a Mock Server in Postman
- Technical requirements
- Different approaches to testing with mocks
- Setting up a mock server
- Creating a mock server from a specification file
- Creating a mock server from a collection
- Creating a new mock server from scratch
- Creating good mock responses
- Using default examples
- Creating custom examples
- Using dynamic variables in examples
- Responding based on parameters
- Understanding example matching
- Using mock servers
- Developing against a mock server
- Continuous planning with a mock server
- Testing with a mock server
- Summary
- Chapter 13: Using Contract Testing to Verify an API
- Understanding contract testing
- What is contract testing?
- How to use contract testing
- Who creates the contracts?
- Setting up contract tests in Postman
- Creating a contract testing collection
- Adding tests to a contract test collection
- Running and fixing contract tests
- Fixing contract test failures
- Sharing contract tests
- Summary
- Chapter 14: Design and Create an API
- Technical requirements
- Designing an API
- Setting up the challenge
- Challenge – Design a usable API
- Solution – Design a usable API
- Challenge 2 – Create an API specification file
- Solution 2 – Create an API specification file
- Setting up the API in Postman
- Challenge – Add an API and mock server in Postman
- Solution – Add an API and mock server in Postman
- Creating tests and documentation for the API
- Challenge – Add tests to the API
- Solution – Add tests to the API
- Sharing what you have done
- Summary