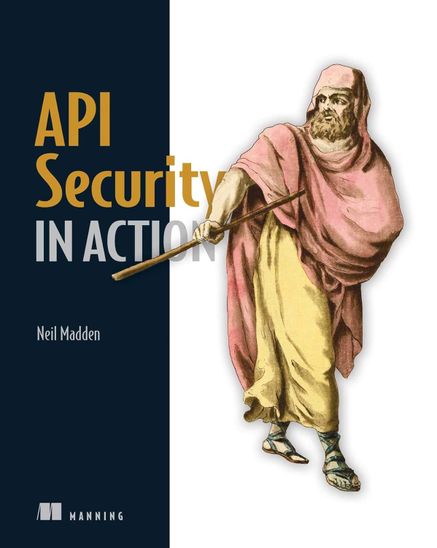
API
Web Security
API Security in Action
Neil Madden, 2021
Inhaltsverzeichnis des Buches
- front matter
- preface
- acknowledgments
- about this book
- Who should read this book
- How this book is organized: A roadmap
- About the code
- liveBook discussion forum
- Other online resources
- about the author
- about the cover illustration
- Part 1. Foundations
- 1 What is API security?
- 1.1 An analogy: Taking your driving test
- 1.2 What is an API?
- 1.2.1 API styles
- 1.3 API security in context
- 1.3.1 A typical API deployment
- 1.4 Elements of API security
- 1.4.1 Assets
- 1.4.2 Security goals
- 1.4.3 Environments and threat models
- 1.5 Security mechanisms
- 1.5.1 Encryption
- 1.5.2 Identification and authentication
- 1.5.3 Access control and authorization
- 1.5.4 Audit logging
- 1.5.5 Rate-limiting
- Answers to pop quiz questions
- Summary
- 2 Secure API development
- 2.1 The Natter API
- 2.1.1 Overview of the Natter API
- 2.1.2 Implementation overview
- 2.1.3 Setting up the project
- 2.1.4 Initializing the database
- 2.2 Developing the REST API
- 2.2.1 Creating a new space
- 2.3 Wiring up the REST endpoints
- 2.3.1 Trying it out
- 2.4 Injection attacks
- 2.4.1 Preventing injection attacks
- 2.4.2 Mitigating SQL injection with permissions
- 2.5 Input validation
- 2.6 Producing safe output
- 2.6.1 Exploiting XSS Attacks
- 2.6.2 Preventing XSS
- 2.6.3 Implementing the protections
- Answers to pop quiz questions
- Summary
- 3 Securing the Natter API
- 3.1 Addressing threats with security controls
- 3.2 Rate-limiting for availability
- 3.2.1 Rate-limiting with Guava
- 3.3 Authentication to prevent spoofing
- 3.3.1 HTTP Basic authentication
- 3.3.2 Secure password storage with Scrypt
- 3.3.3 Creating the password database
- 3.3.4 Registering users in the Natter API
- 3.3.5 Authenticating users
- 3.4 Using encryption to keep data private
- 3.4.1 Enabling HTTPS
- 3.4.2 Strict transport security
- 3.5 Audit logging for accountability
- 3.6 Access control
- 3.6.1 Enforcing authentication
- 3.6.2 Access control lists
- 3.6.3 Enforcing access control in Natter
- 3.6.4 Adding new members to a Natter space
- 3.6.5 Avoiding privilege escalation attacks
- Answers to pop quiz questions
- Summary
- Part 2. Token-based authentication
- 4 Session cookie authentication
- 4.1 Authentication in web browsers
- 4.1.1 Calling the Natter API from JavaScript
- 4.1.2 Intercepting form submission
- 4.1.3 Serving the HTML from the same origin
- 4.1.4 Drawbacks of HTTP authentication
- 4.2 Token-based authentication
- 4.2.1 A token store abstraction
- 4.2.2 Implementing token-based login
- 4.3 Session cookies
- 4.3.1 Avoiding session fixation attacks
- 4.3.2 Cookie security attributes
- 4.3.3 Validating session cookies
- 4.4 Preventing Cross-Site Request Forgery attacks
- 4.4.1 SameSite cookies
- 4.4.2 Hash-based double-submit cookies
- 4.4.3 Double-submit cookies for the Natter API
- 4.5 Building the Natter login UI
- 4.5.1 Calling the login API from JavaScript
- 4.6 Implementing logout
- Answers to pop quiz questions
- Summary
- 5 Modern token-based authentication
- 5.1 Allowing cross-domain requests with CORS
- 5.1.1 Preflight requests
- 5.1.2 CORS headers
- 5.1.3 Adding CORS headers to the Natter API
- 5.2 Tokens without cookies
- 5.2.1 Storing token state in a database
- 5.2.2 The Bearer authentication scheme
- 5.2.3 Deleting expired tokens
- 5.2.4 Storing tokens in Web Storage
- 5.2.5 Updating the CORS filter
- 5.2.6 XSS attacks on Web Storage
- 5.3 Hardening database token storage
- 5.3.1 Hashing database tokens
- 5.3.2 Authenticating tokens with HMAC
- 5.3.3 Protecting sensitive attributes
- Answers to pop quiz questions
- Summary
- 6 Self-contained tokens and JWTs
- 6.1 Storing token state on the client
- 6.1.1 Protecting JSON tokens with HMAC
- 6.2 JSON Web Tokens
- 6.2.1 The standard JWT claims
- 6.2.2 The JOSE header
- 6.2.3 Generating standard JWTs
- 6.2.4 Validating a signed JWT
- 6.3 Encrypting sensitive attributes
- 6.3.1 Authenticated encryption
- 6.3.2 Authenticated encryption with NaCl
- 6.3.3 Encrypted JWTs
- 6.3.4 Using a JWT library
- 6.4 Using types for secure API design
- 6.5 Handling token revocation
- 6.5.1 Implementing hybrid tokens
- Answers to pop quiz questions
- Summary
- Part 3. Authorization
- 7 OAuth2 and OpenID Connect
- 7.1 Scoped tokens
- 7.1.1 Adding scoped tokens to Natter
- 7.1.2 The difference between scopes and permissions
- 7.2 Introducing OAuth2
- 7.2.1 Types of clients
- 7.2.2 Authorization grants
- 7.2.3 Discovering OAuth2 endpoints
- 7.3 The Authorization Code grant
- 7.3.1 Redirect URIs for different types of clients
- 7.3.2 Hardening code exchange with PKCE
- 7.3.3 Refresh tokens
- 7.4 Validating an access token
- 7.4.1 Token introspection
- 7.4.2 Securing the HTTPS client configuration
- 7.4.3 Token revocation
- 7.4.4 JWT access tokens
- 7.4.5 Encrypted JWT access tokens
- 7.4.6 Letting the AS decrypt the tokens
- 7.5 Single sign-on
- 7.6 OpenID Connect
- 7.6.1 ID tokens
- 7.6.2 Hardening OIDC
- 7.6.3 Passing an ID token to an API
- Answers to pop quiz questions
- Summary
- 8 Identity-based access control
- 8.1 Users and groups
- 8.1.1 LDAP groups
- 8.2 Role-based access control
- 8.2.1 Mapping roles to permissions
- 8.2.2 Static roles
- 8.2.3 Determining user roles
- 8.2.4 Dynamic roles
- 8.3 Attribute-based access control
- 8.3.1 Combining decisions
- 8.3.2 Implementing ABAC decisions
- 8.3.3 Policy agents and API gateways
- 8.3.4 Distributed policy enforcement and XACML
- 8.3.5 Best practices for ABAC
- Answers to pop quiz questions
- Summary
- 9 Capability-based security and macaroons
- 9.1 Capability-based security
- 9.2 Capabilities and REST
- 9.2.1 Capabilities as URIs
- 9.2.2 Using capability URIs in the Natter API
- 9.2.3 HATEOAS
- 9.2.4 Capability URIs for browser-based clients
- 9.2.5 Combining capabilities with identity
- 9.2.6 Hardening capability URIs
- 9.3 Macaroons: Tokens with caveats
- 9.3.1 Contextual caveats
- 9.3.2 A macaroon token store
- 9.3.3 First-party caveats
- 9.3.4 Third-party caveats
- Answers to pop quiz questions
- Summary
- Part 4. Microservice APIs in Kubernetes
- 10 Microservice APIs in Kubernetes
- 10.1 Microservice APIs on Kubernetes
- 10.2 Deploying Natter on Kubernetes
- 10.2.1 Building H2 database as a Docker container
- 10.2.2 Deploying the database to Kubernetes
- 10.2.3 Building the Natter API as a Docker container
- 10.2.4 The link-preview microservice
- 10.2.5 Deploying the new microservice
- 10.2.6 Calling the link-preview microservice
- 10.2.7 Preventing SSRF attacks
- 10.2.8 DNS rebinding attacks
- 10.3 Securing microservice communications
- 10.3.1 Securing communications with TLS
- 10.3.2 Using a service mesh for TLS
- 10.3.3 Locking down network connections
- 10.4 Securing incoming requests
- Answers to pop quiz questions
- Summary
- 11 Securing service-to-service APIs
- 11.1 API keys and JWT bearer authentication
- 11.2 The OAuth2 client credentials grant
- 11.2.1 Service accounts
- 11.3 The JWT bearer grant for OAuth2
- 11.3.1 Client authentication
- 11.3.2 Generating the JWT
- 11.3.3 Service account authentication
- 11.4 Mutual TLS authentication
- 11.4.1 How TLS certificate authentication works
- 11.4.2 Client certificate authentication
- 11.4.3 Verifying client identity
- 11.4.4 Using a service mesh
- 11.4.5 Mutual TLS with OAuth2
- 11.4.6 Certificate-bound access tokens
- 11.5 Managing service credentials
- 11.5.1 Kubernetes secrets
- 11.5.2 Key and secret management services
- 11.5.3 Avoiding long-lived secrets on disk
- 11.5.4 Key derivation
- 11.6 Service API calls in response to user requests
- 11.6.1 The phantom token pattern
- 11.6.2 OAuth2 token exchange
- Answers to pop quiz questions
- Summary
- Part 5. APIs for the Internet of Things
- 12 Securing IoT communications
- 12.1 Transport layer security
- 12.1.1 Datagram TLS
- 12.1.2 Cipher suites for constrained devices
- 12.2 Pre-shared keys
- 12.2.1 Implementing a PSK server
- 12.2.2 The PSK client
- 12.2.3 Supporting raw PSK cipher suites
- 12.2.4 PSK with forward secrecy
- 12.3 End-to-end security
- 12.3.1 COSE
- 12.3.2 Alternatives to COSE
- 12.3.3 Misuse-resistant authenticated encryption
- 12.4 Key distribution and management
- 12.4.1 One-off key provisioning
- 12.4.2 Key distribution servers
- 12.4.3 Ratcheting for forward secrecy
- 12.4.4 Post-compromise security
- Answers to pop quiz questions
- Summary
- 13 Securing IoT APIs
- 13.1 Authenticating devices
- 13.1.1 Identifying devices
- 13.1.2 Device certificates
- 13.1.3 Authenticating at the transport layer
- 13.2 End-to-end authentication
- 13.2.1 OSCORE
- 13.2.2 Avoiding replay in REST APIs
- 13.3 OAuth2 for constrained environments
- 13.3.1 The device authorization grant
- 13.3.2 ACE-OAuth
- 13.4 Offline access control
- 13.4.1 Offline user authentication
- 13.4.2 Offline authorization
- Answers to pop quiz questions
- Summary
- appendix A. Setting up Java and Maven
- appendix B. Setting up Kubernetes
- index